- inputsThe input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given.
C++ Type:std::vector<MeshGeneratorName>
Controllable:No
Description:The input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given.
CombinerGenerator
Combine multiple meshes (or copies of one mesh) together into one (disjoint) mesh. Can optionally translate those meshes before combining them.
Overview
The CombinerGenerator
allows the user to combine the outputs of multiple MeshGenerator
s into a single mesh. This is somewhat similar to the StitchedMeshGenerator with the difference being that CombinerGenerator
makes no attempt to "heal" / "join" the mesh like StitchedMeshGenerator does. There CombinerGenerator
is more suited to creation of disjoint meshes (where the individual pieces are not directly tied together).
CombinerGenerator
preserves subdomain names and boundary names (node sets, side sets, and edge sets). If the corresponding IDs exist in multiple meshes, then the meshes/copies listed later in "inputs"/"positions" take precedence.
Usage
There are three main ways to use the CombinerGenerator
:
1. Combine Multiple MeshGenerator
s
The most straightforward thing to do is simply to combine the output of multiple MeshGenerator
s together into a single mesh. For example:
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[gen1]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 10
ny<<<{"description": "Number of elements in the Y direction"}>>> = 10
xmin<<<{"description": "Lower X Coordinate of the generated mesh"}>>> = 0
xmax<<<{"description": "Upper X Coordinate of the generated mesh"}>>> = 1
ymin<<<{"description": "Lower Y Coordinate of the generated mesh"}>>> = 0
ymax<<<{"description": "Upper Y Coordinate of the generated mesh"}>>> = 1
[]
[gen2]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 12
ny<<<{"description": "Number of elements in the Y direction"}>>> = 12
xmin<<<{"description": "Lower X Coordinate of the generated mesh"}>>> = 2
xmax<<<{"description": "Upper X Coordinate of the generated mesh"}>>> = 3
ymin<<<{"description": "Lower Y Coordinate of the generated mesh"}>>> = 2
ymax<<<{"description": "Upper Y Coordinate of the generated mesh"}>>> = 3
[]
[gen3]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 14
ny<<<{"description": "Number of elements in the Y direction"}>>> = 14
xmin<<<{"description": "Lower X Coordinate of the generated mesh"}>>> = 3.5
xmax<<<{"description": "Upper X Coordinate of the generated mesh"}>>> = 5
ymin<<<{"description": "Lower Y Coordinate of the generated mesh"}>>> = 3
ymax<<<{"description": "Upper Y Coordinate of the generated mesh"}>>> = 4
[]
[cmbn]
type = CombinerGenerator<<<{"description": "Combine multiple meshes (or copies of one mesh) together into one (disjoint) mesh. Can optionally translate those meshes before combining them.", "href": "CombinerGenerator.html"}>>>
inputs<<<{"description": "The input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given."}>>> = 'gen1 gen2 gen3'
[]
[]
(test/tests/meshgenerators/combiner_generator/combiner_multi_input.i)Will generate a mesh that looks like:
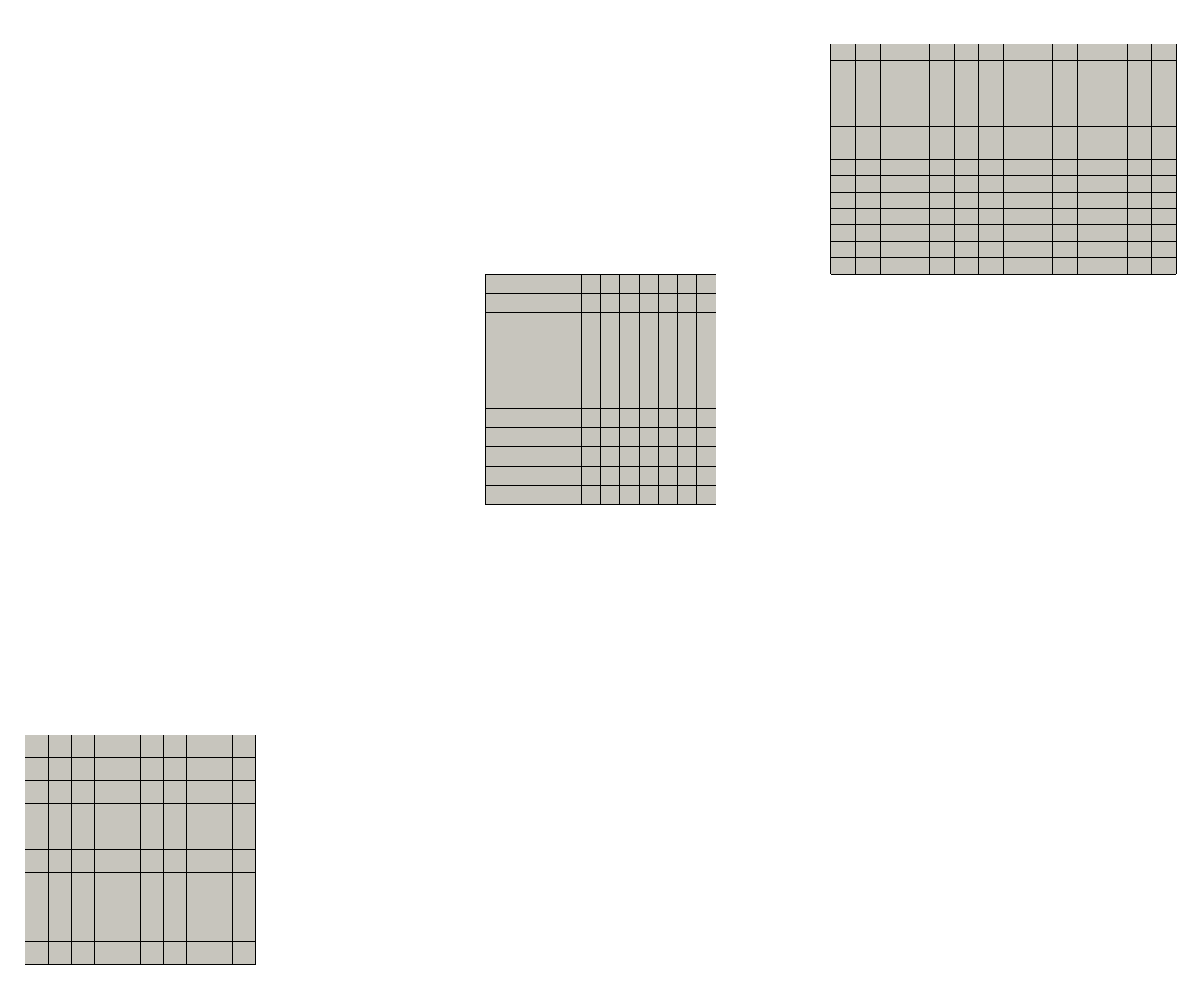
2. Combine Multiple MeshGenerator
s AND Translate Them
It is also possible to translate (move) the input MeshGenerator
s as they are combined. This is done using the positions
option which takes triplets of floating point numbers that are interpreted as displacement vectors for moving each of the input meshes.
If you specify positions
then the number of positions
must match the number of inputs
, unless only one input is specified (more on that in a moment).
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[gen1]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 10
ny<<<{"description": "Number of elements in the Y direction"}>>> = 10
[]
[gen2]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 12
ny<<<{"description": "Number of elements in the Y direction"}>>> = 12
[]
[gen3]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 14
ny<<<{"description": "Number of elements in the Y direction"}>>> = 14
[]
[cmbn]
type = CombinerGenerator<<<{"description": "Combine multiple meshes (or copies of one mesh) together into one (disjoint) mesh. Can optionally translate those meshes before combining them.", "href": "CombinerGenerator.html"}>>>
inputs<<<{"description": "The input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given."}>>> = 'gen1 gen2 gen3'
positions<<<{"description": "The (optional) position of each given mesh. If N 'inputs' were given then this must either be left blank or N positions must be given. If 1 input was given then this MUST be provided."}>>> = '1 0 0 2 2 2 3 0 0'
[]
[]
(test/tests/meshgenerators/combiner_generator/combiner_multi_input_translate.i)Will generate a mesh that looks like:
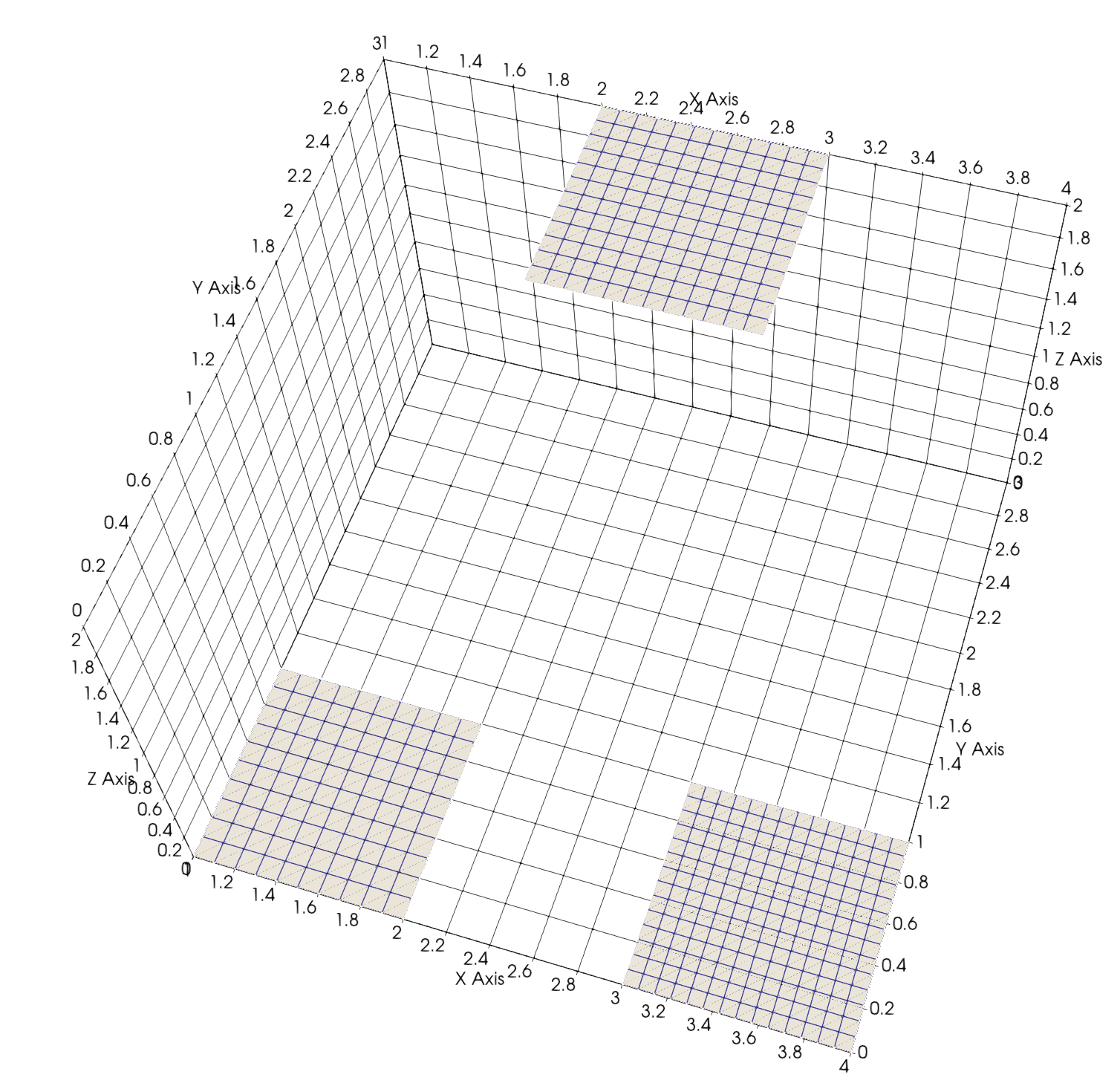
Alternatively, the same displacement vectors can be supplied in a file with the positions_file
option. The above mesh can equivalently be generated with the following.
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[gen1]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 10
ny<<<{"description": "Number of elements in the Y direction"}>>> = 10
[]
[gen2]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 12
ny<<<{"description": "Number of elements in the Y direction"}>>> = 12
[]
[gen3]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 14
ny<<<{"description": "Number of elements in the Y direction"}>>> = 14
[]
[cmbn]
type = CombinerGenerator<<<{"description": "Combine multiple meshes (or copies of one mesh) together into one (disjoint) mesh. Can optionally translate those meshes before combining them.", "href": "CombinerGenerator.html"}>>>
inputs<<<{"description": "The input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given."}>>> = 'gen1 gen2 gen3'
positions_file<<<{"description": "Alternative way to provide the position of each given mesh."}>>> = 'positions.txt'
[]
[]
(test/tests/meshgenerators/combiner_generator/combiner_multi_input_translate_from_file.i)where the positions.txt
file contains the floating point triplets.
1 0 0
2 2 2
3 0 0
(test/tests/meshgenerators/combiner_generator/positions.txt)The same restrictions on positions
also apply to the number of entries in position_file
.
3. Copy a Single Input Multiple Times With Translations
The final option is to provide exactly one inputs
but specify multiple positions
. This will cause the single input to be copied multiple times with the position of each copy specified by the positions
parameter. For example
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[gen]
type = GeneratedMeshGenerator<<<{"description": "Create a line, square, or cube mesh with uniformly spaced or biased elements.", "href": "GeneratedMeshGenerator.html"}>>>
dim<<<{"description": "The dimension of the mesh to be generated"}>>> = 2
nx<<<{"description": "Number of elements in the X direction"}>>> = 10
ny<<<{"description": "Number of elements in the Y direction"}>>> = 10
[]
[cmbn]
type = CombinerGenerator<<<{"description": "Combine multiple meshes (or copies of one mesh) together into one (disjoint) mesh. Can optionally translate those meshes before combining them.", "href": "CombinerGenerator.html"}>>>
inputs<<<{"description": "The input MeshGenerators. This can either be N generators or 1 generator. If only 1 is given then 'positions' must also be given."}>>> = 'gen'
positions<<<{"description": "The (optional) position of each given mesh. If N 'inputs' were given then this must either be left blank or N positions must be given. If 1 input was given then this MUST be provided."}>>> = '1 0 0 2 2 2 3 0 0'
[]
[]
(test/tests/meshgenerators/combiner_generator/combiner_generator.i)Will generate a mesh that looks like:
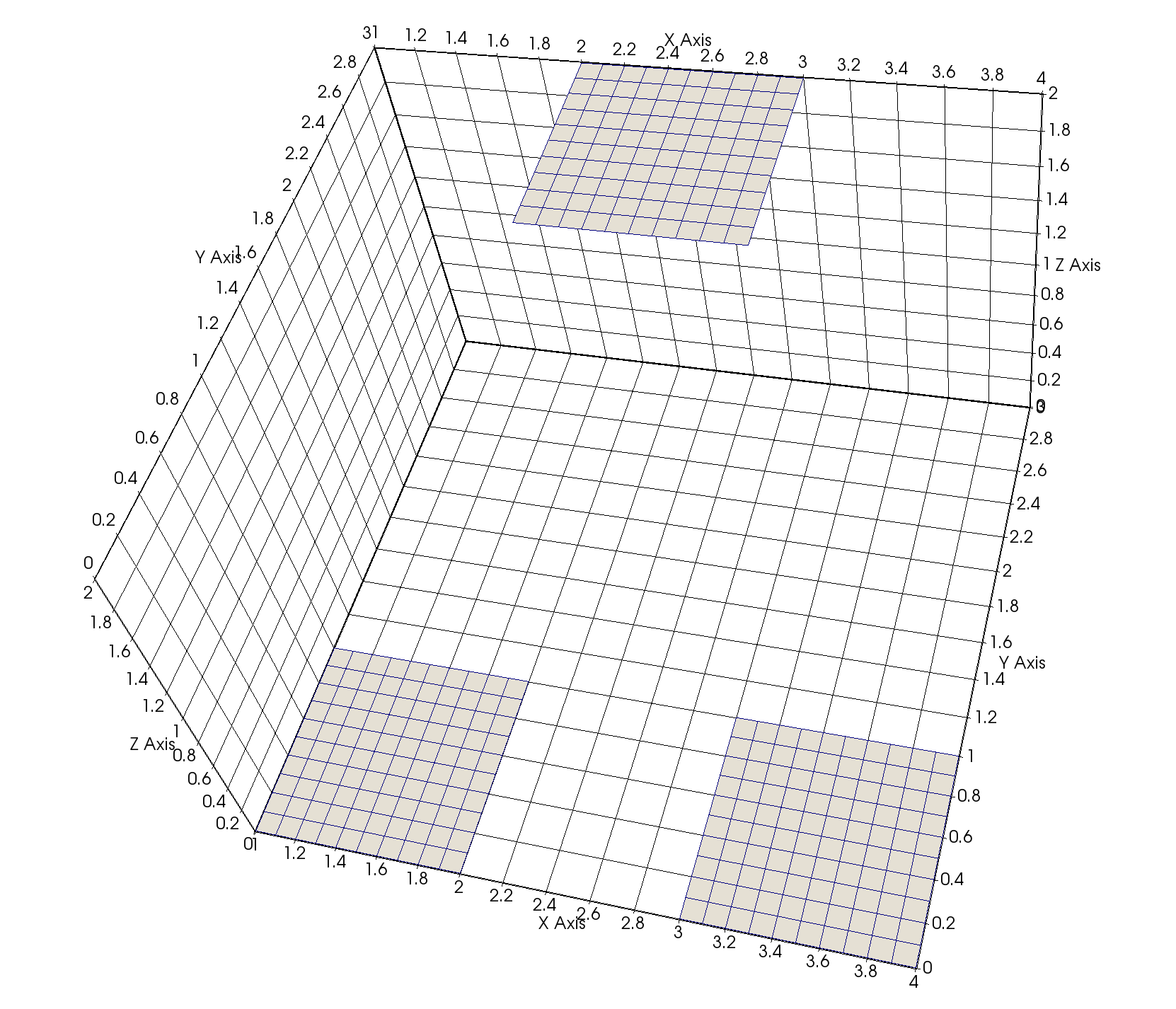
Again, the same capability can be achieved with the positions_file
option.
Input Parameters
- avoid_merging_boundariesFalseWhether to prevent merging sidesets by offsetting ids. The first mesh in the input will keep the same boundary ids, the others will have offsets. All boundary names will remain valid
Default:False
C++ Type:bool
Controllable:No
Description:Whether to prevent merging sidesets by offsetting ids. The first mesh in the input will keep the same boundary ids, the others will have offsets. All boundary names will remain valid
- avoid_merging_subdomainsFalseWhether to prevent merging subdomains by offsetting ids. The first mesh in the input will keep the same subdomains ids, the others will have offsets. All subdomain names will remain valid
Default:False
C++ Type:bool
Controllable:No
Description:Whether to prevent merging subdomains by offsetting ids. The first mesh in the input will keep the same subdomains ids, the others will have offsets. All subdomain names will remain valid
- positionsThe (optional) position of each given mesh. If N 'inputs' were given then this must either be left blank or N positions must be given. If 1 input was given then this MUST be provided.
C++ Type:std::vector<libMesh::Point>
Controllable:No
Description:The (optional) position of each given mesh. If N 'inputs' were given then this must either be left blank or N positions must be given. If 1 input was given then this MUST be provided.
- positions_fileAlternative way to provide the position of each given mesh.
C++ Type:std::vector<FileName>
Controllable:No
Description:Alternative way to provide the position of each given mesh.
Optional Parameters
- enableTrueSet the enabled status of the MooseObject.
Default:True
C++ Type:bool
Controllable:No
Description:Set the enabled status of the MooseObject.
- save_with_nameKeep the mesh from this mesh generator in memory with the name specified
C++ Type:std::string
Controllable:No
Description:Keep the mesh from this mesh generator in memory with the name specified
Advanced Parameters
- nemesisFalseWhether or not to output the mesh file in the nemesisformat (only if output = true)
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to output the mesh file in the nemesisformat (only if output = true)
- outputFalseWhether or not to output the mesh file after generating the mesh
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to output the mesh file after generating the mesh
- show_infoFalseWhether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)
Debugging Parameters
Input Files
- (test/tests/meshgenerators/batch_mesh_generation_action/double_vector_cp.i)
- (test/tests/meshgenerators/combiner_generator/combiner_multi_input_translate.i)
- (modules/heat_transfer/test/tests/directional_flux_bc/2d_elem.i)
- (test/tests/meshgenerators/coarsen_block_generator/coarsen_hex.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/single_vector.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/scalar_vector_corr.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/scalar_vector_cp.i)
- (modules/solid_mechanics/test/tests/umat/multiple_blocks/multiple_blocks_two_materials_parallel.i)
- (modules/heat_transfer/test/tests/thermal_materials/2d.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/single_scalar.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/double_vector_corr.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/vector_point.i)
- (modules/contact/test/tests/verification/patch_tests/automatic_patch_update/iteration_adaptivity_parallel.i)
- (test/tests/postprocessors/nearest_node_number/nearest_node_number_2.i)
- (test/tests/meshgenerators/combiner_generator/combiner_generator.i)
- (test/tests/meshgenerators/elements_to_tetrahedrons_convertor/prism_elems.i)
- (modules/contact/test/tests/verification/patch_tests/automatic_patch_update/iteration_adaptivity_parallel_node_face.i)
- (test/tests/meshgenerators/mesh_diagnostics_generator/elem_types_test.i)
- (test/tests/meshgenerators/mesh_repair_generator/overlapping_fix_test.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/mfname.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/double_scalar_corr.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/real_vector_value_mixed.i)
- (test/tests/meshgenerators/combiner_generator/combiner_multi_input_translate_from_file.i)
- (test/tests/meshgenerators/xy_delaunay_generator/xydelaunay_from_2d_bcids.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/scalar_enum_test.i)
- (test/tests/meshgenerators/mesh_diagnostics_generator/detect_amr_hex.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/double_scalar_cp.i)
- (test/tests/meshgenerators/combiner_generator/combiner_merge_names.i)
- (test/tests/meshgenerators/elem_order_conversion_generator/order_conversion.i)
- (modules/heat_transfer/test/tests/directional_flux_bc/3d_elem.i)
- (test/tests/meshgenerators/mesh_diagnostics_generator/node_based_test.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/real_vector_value.i)
- (test/tests/mortar/coincident-nodes/test.i)
- (test/tests/meshgenerators/coarsen_block_generator/coarsen_quad.i)
- (test/tests/meshgenerators/elements_to_tetrahedrons_convertor/pyramid_elems.i)
- (modules/reactor/test/tests/meshgenerators/azimuthal_block_split_generator/err_mixed_order.i)
- (test/tests/meshgenerators/xy_delaunay_generator/xydelaunay_from_1d_sbdids.i)
- (modules/solid_mechanics/test/tests/umat/multiple_blocks/multiple_blocks.i)
- (modules/optimization/test/tests/simp/2d.i)
- (test/tests/meshgenerators/elements_to_tetrahedrons_convertor/hex_elems.i)
- (test/tests/coord_type/coord_type_rz_general.i)
- (test/tests/meshgenerators/combiner_generator/combiner_generator_from_file.i)
- (test/tests/mesh/blocks_max_dimension/blocks_max_dimension.i)
- (modules/heat_transfer/test/tests/gap_perfect_transfer/perfect_transfer_gap.i)
- (modules/heat_transfer/test/tests/gap_heat_transfer_radiation/cylinder.i)
- (test/tests/meshgenerators/mesh_repair_generator/mixed_elements.i)
- (modules/heat_transfer/test/tests/directional_flux_bc/3d.i)
- (modules/solid_mechanics/test/tests/umat/multiple_blocks/multiple_blocks_two_materials.i)
- (test/tests/meshgenerators/mesh_diagnostics_generator/detect_amr_quad.i)
- (modules/heat_transfer/test/tests/directional_flux_bc/2d.i)
- (test/tests/meshgenerators/combiner_generator/combiner_multi_input.i)
- (modules/optimization/test/tests/simp/2d_twoconstraints.i)
- (modules/heat_transfer/test/tests/gap_heat_transfer_htonly/1D.i)
- (modules/heat_transfer/test/tests/gap_heat_transfer_radiation/sphere.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/mg_sd_name.i)
- (test/tests/meshgenerators/parsed_element_deletion_generator/cut_the_small.i)
- (test/tests/meshgenerators/mesh_repair_generator/flip_element.i)
- (test/tests/meshgenerators/batch_mesh_generation_action/mg_bdry_name.i)